Today at work, we came across an interesting bug while debugging some code. We accidentally tried to access a linked list element using an index, as if it were an array. At first, the code seemed fine—it compiled without any errors. This was because the linked list was part of a structure defined as a pointer of pointers. However, the code didn’t work as expected when we ran it.
This experience reminded us how important it is to really understand how the tools we use actually work. Writing code that runs is good, but knowing why it works (or doesn’t) is even more important.
In this blog post, I’ll share what happened, explain the difference between arrays and linked lists, and talk about what we missed and why and how. Hopefully, it will help others avoid the same mistake and better understand these basic data structures.
Let’s start with a simple example.
What do you think about the memory allocation at this point after we run this code above? Arrays are stored in consecutive memory addresses, while linked lists organize their elements non-sequentially (it does not have to be, but it also might be). Each node in a linked list includes a pointer that links it to the following node. Let’s see the memory allocation in a visual way:
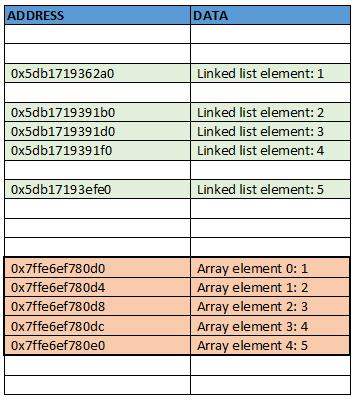
Let’s talk about accessing the data in those structures…
In C, we all know, accessing an array element by its index is straightforward because arrays are stored in contiguous memory locations. However, linked lists do not store their elements contiguously; each element (node) contains a pointer to the next element. Therefore, you cannot access a linked list element directly by an index like you can with an array.
If you try to access a linked list element using an index (e.g., head[index]
), it will result in a compilation error because the head
pointer is not an array, and the indexing operator []
is not defined for linked list nodes. To access an element at a specific position in a linked list, you need to traverse the list from the head node to the desired position.
Then why we did not get any compilation error while trying to access linked list elements by index?
Let’s imagine a new structure that contains a pointer to a linked list (head). Here is an example:
As you can see here, everything is almost the same, except new “SomeClass” and its object “myObject”. Since we have a pointer to linked list in “myObject”… it’s easy to get confused about it. As shown in the code, if you try to access the data by index, the compilation will be OK, but your program does not run as expected, it will lead to random values or crashes. Because…
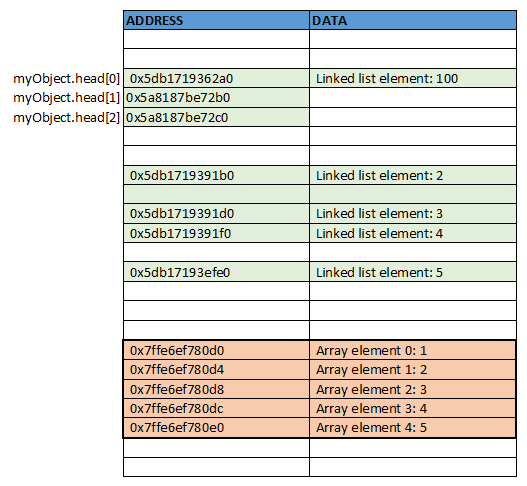
What is the correct way?
To correctly access an element at a specific index in a linked list, you need to traverse the list from the head node to the desired position… something like this:
// Update the data of the linked list nodes
myObject.head->data = 100;
myObject.head->next->data = 200;
myObject.head->next->next->data = 300;
///////// OR /////////
// Function to get the data of the node at a specific index in the linked list
int getNodeDataAtIndex(struct Node* head, int index) {
struct Node* temp = head;
int count = 0;
// Traverse the list until the desired index is reached
while (temp != NULL) {
if (count == index) {
return temp->data;
}
count++;
temp = temp->next;
}
// If the index is out of bounds, return -1 or handle the error as needed
printf("Index out of bounds\n");
return -1;
}
Leave a Reply