A long time since I posted the last time… I have been studying new topics. Today, I’d like to share a post about a DSP function on ARM processors. It is “convolution.”
Let’s begin with the definition. I can say that the magic keyword behind the convolution is “signal decomposition”. You should investigate their relationship. Also, you can also check out this chapter or this series. They describe the connection between impulse response and convolution function in a nutshell. I merely provide you with the source because I don’t have enough time to explain them from scratch or to make them animated.
Here is the discrete equation of the convolution:
-point signal from 0 to
:
-point signal from 0 to
-1
point signal from 0 to
Let’s analyze a specific output point, how does it calculated…
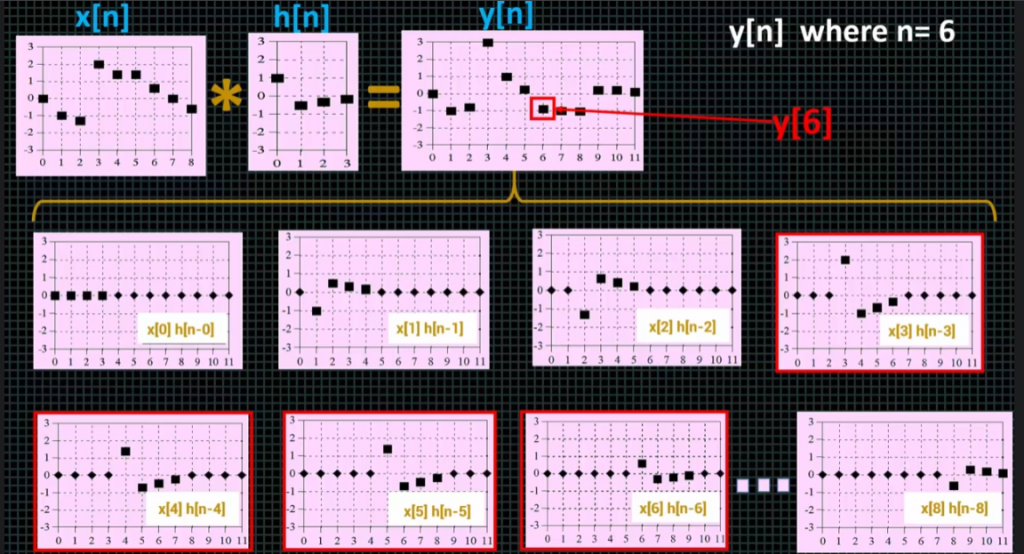
As can be seen from the explanation above, we can decompose the output signal to decide which scale/shift step of impulse response affects the 6th element at the output. The pattern can be seen clearly!
So, the sum of i and j values which are index values of the input signal and the impulse response must be the same as the index value of the output signal which we’re analyzing. It is our algorithm…
The signals on the logic analyzer:
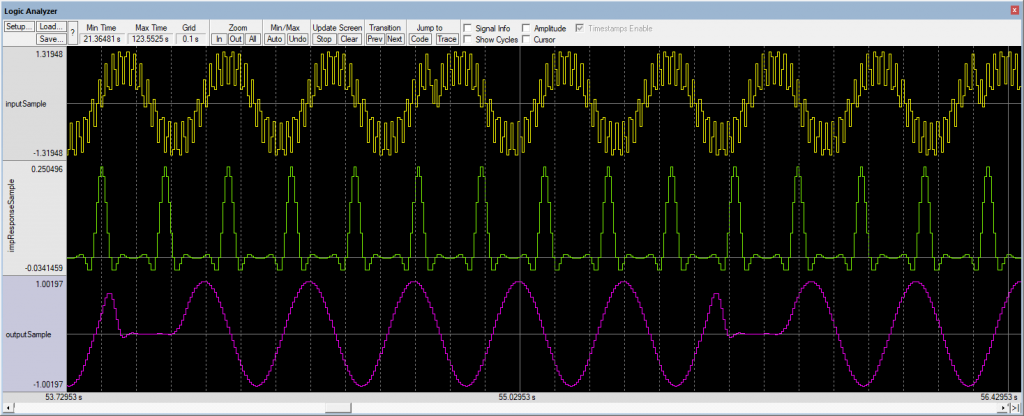
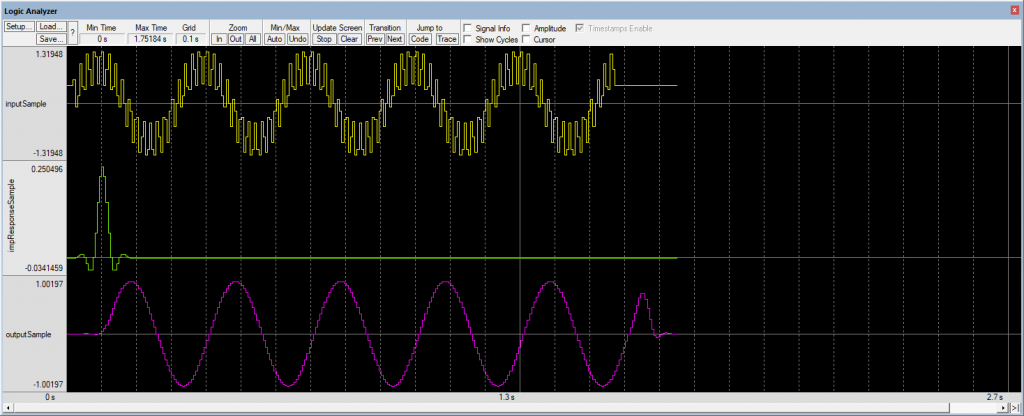
It’s the first part of the post… CMSIS Convolution will be described and implemented in the next post…
I am waiting for your feedback… tips and tricks, or my faults, etc.
Leave a Reply